论文地址:deep residue network
CIFAR-10 dataset
CIFAR-10 数据集包含60000幅大小为 32 x 32
的彩色图像,这些图像分别属于10个类,每个类 6000 幅图像。数据集分为5个训练batch和1个测试batch,每个batch包含 10000 幅图像。
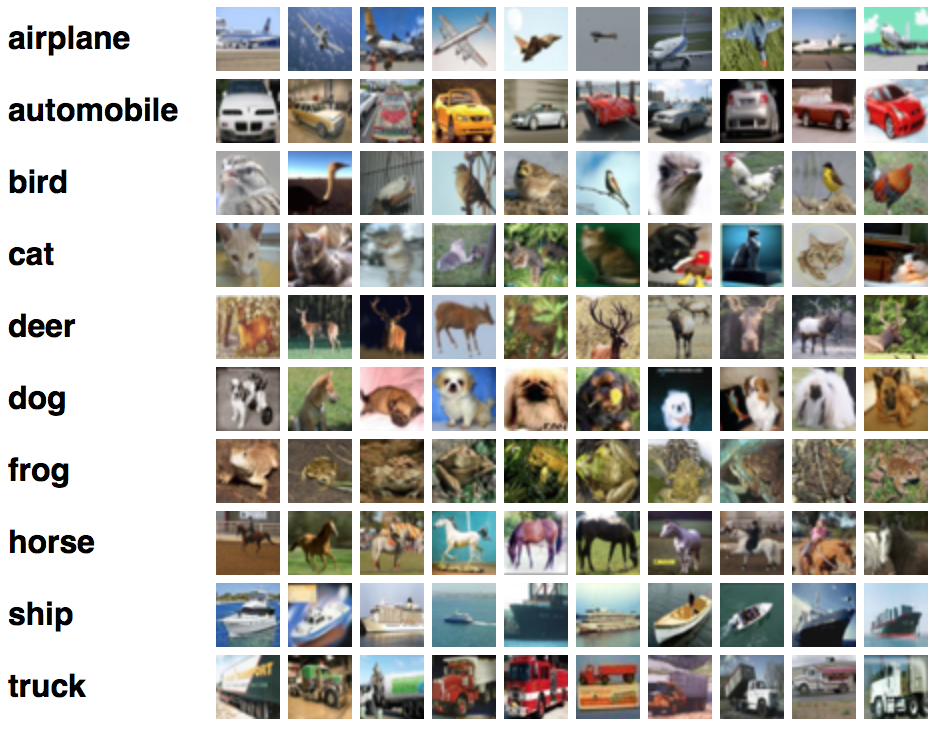
数据准备
下载 CIFAR-10
打开终端,在
$CAFFE_ROOT
(caffe 的根目录)下输入命令:
|
|
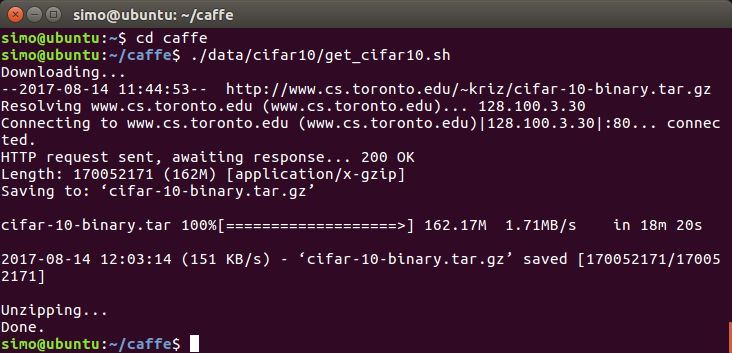
下载完成后,会在 $CAFFE_ROOT/data/cifar10/
中生成数据 batch,不过这些都是二进制文件,需要转换为 LMDB 格式。
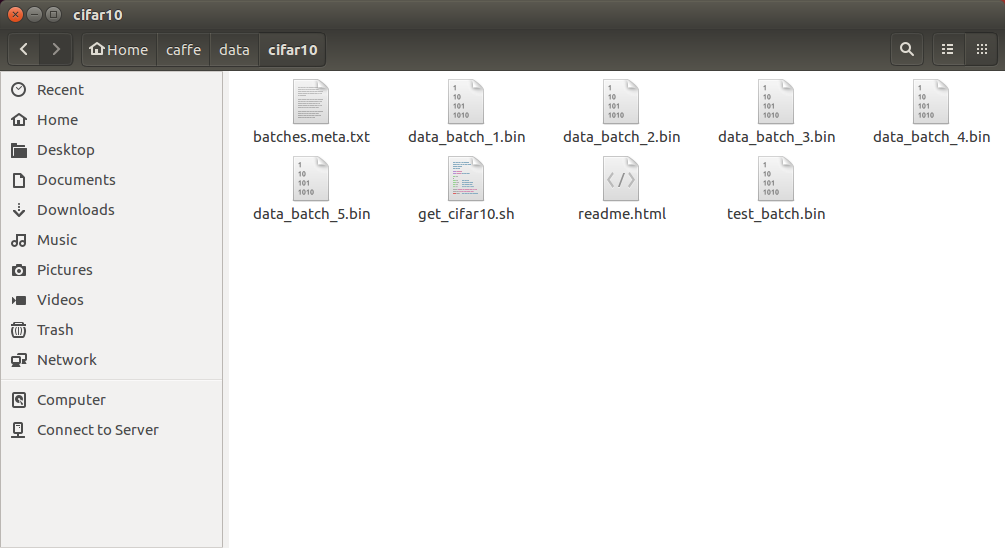
- 生成 LMDB 文件
|
|
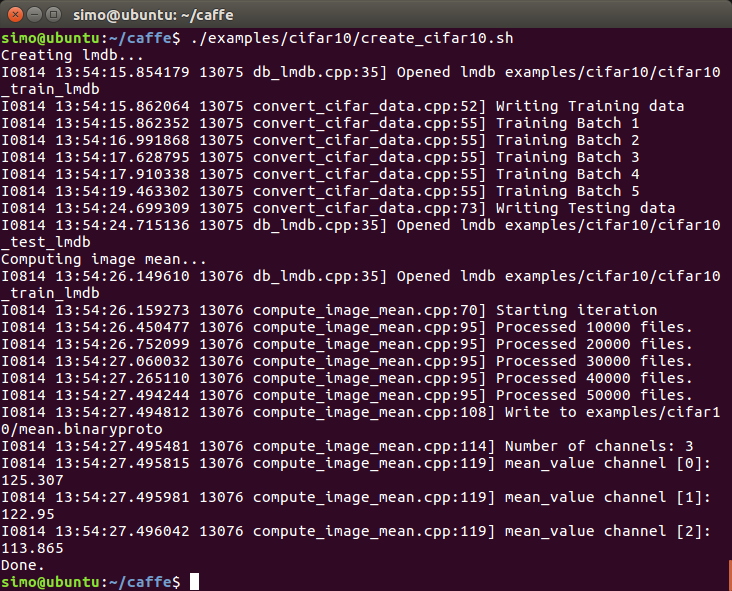
这样就生成了 LMDB 文件,同时生成了训练数据 (cifar10_train_lmdb
) 的均值文件 mean.binaryproto
。均值文件的计算方式是计算不同通道上的样本均值,在 CIFAR-10 中,训练数据 size 为 5000 x 3 x 32 x 32
(样本数 x 图像通道数 x 图像高度 x 图像宽度),则均值的 size 是 3
。
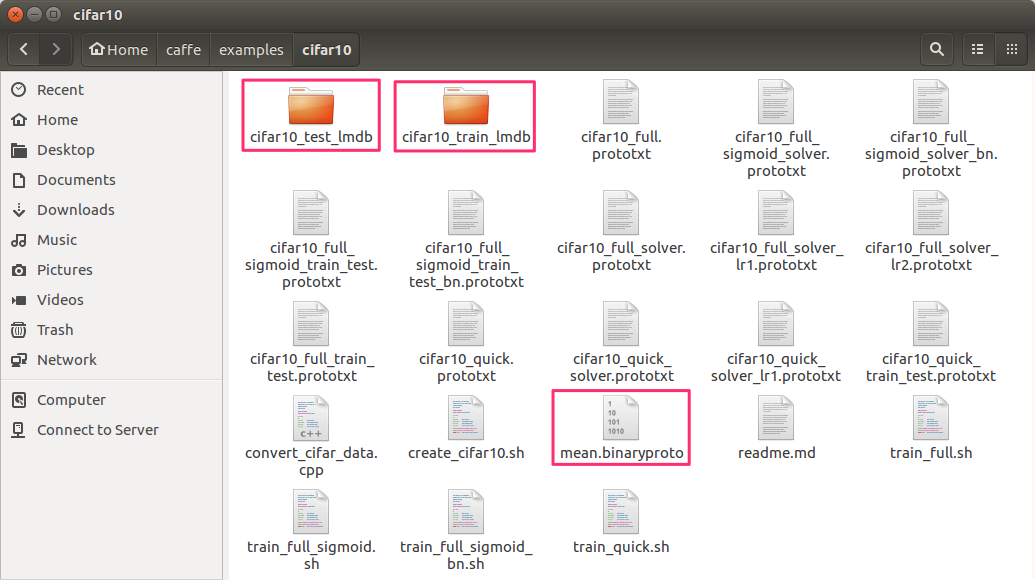
搭建网络
在 $CAFFE_ROOT/examples/
目录下新建文件夹 ResNet
,并在文件夹下新建 resnet_cifar10.py
.
import modules
|
|
网络层函数
ResNet 中两个基本的结构如下:
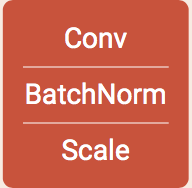
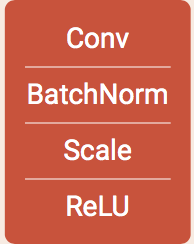
定义层函数:
|
|
残差模块
ResNet 论文中在 CIFAR-10 数据集上实验采用的残差模块由两层 3x3 卷积加一个 skip connection构成:
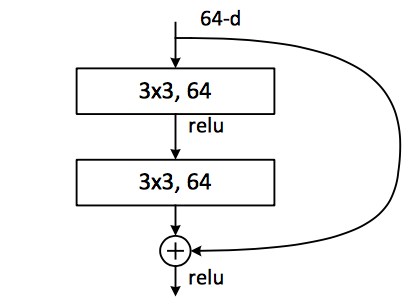
定义残差模块:
|
|
ResNet
论文中定义在 CIFAR-10 数据集上实验的 ResNet 结构为:
layer name | output size | ResNet |
---|---|---|
conv1 | 32x32 | 3x3, 16, stride 1 |
conv2_x | 32x32 | $$\left[ \begin{matrix} 3\times3, 16 \ 3\times3, 16 \end{matrix} \right] \times n$$ |
conv3_x | 16x16 | $$\left[ \begin{matrix} 3\times3, 32 \ 3\times3, 32 \end{matrix} \right] \times n$$ |
conv4_x | 8x8 | $$\left[ \begin{matrix} 3\times3, 64 \ 3\times3, 64 \end{matrix} \right] \times n$$ |
pool4 | 1x1 | average pool |
fc10 | 10 | 10-d fc |
定义ResNet网络:
|
|
将网络结构写入 prototxt
文件
|
|
生成 solver.prototxt
文件
添加 tools 模块
|
|
修改 tools.py
由于采用 multistep
学习策略时,可能有多个 stepvalue
值,若直接以字典赋值会覆盖,故修改 tools.py
文件的 write()
函数。
|
|
写入 solver.prototxt
|
|
执行训练
在 $CAFFE_ROOT
目录下运行:
|
|